Here is the config ..
Intel Celeron M 360 (some 1.4 or 1.6GHz, forgot)
256MB Ram, 40GB Harddisk, 15" screen and a bunch of other crap ..
I always wanted a small laptop (a 12" .. it looks so cool), but unfortunately i got a 15" (as i said, i went for the cheapest one) .. its slightly bigger, and well, doesnt look good on lap. Also screen is pathetic (i didnt notice the laptop screens much before this) .. the laptop screen tends to have a bluish tint with a little bit of gamma.. (i checked with the thinkpad here in office)
It came with some Lupus linux (hell it didnt even have GUI) .. i tried installing Fedora Core, but its identifying as VESA .. i need to find the proper drivers ..
Graphics is pretty ok .. it gives around 80fps in 640x480 quake3 and is playable (40 fps i guess) in 1024x728 with high settings .. but again, the screen makes it pathetic to play with (it has upto 128MB shared mem)
All in all its an okay system, comparing the price.. it cost me 32k with carry bag (damn im bankrupt again) ..
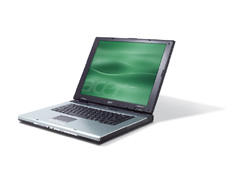